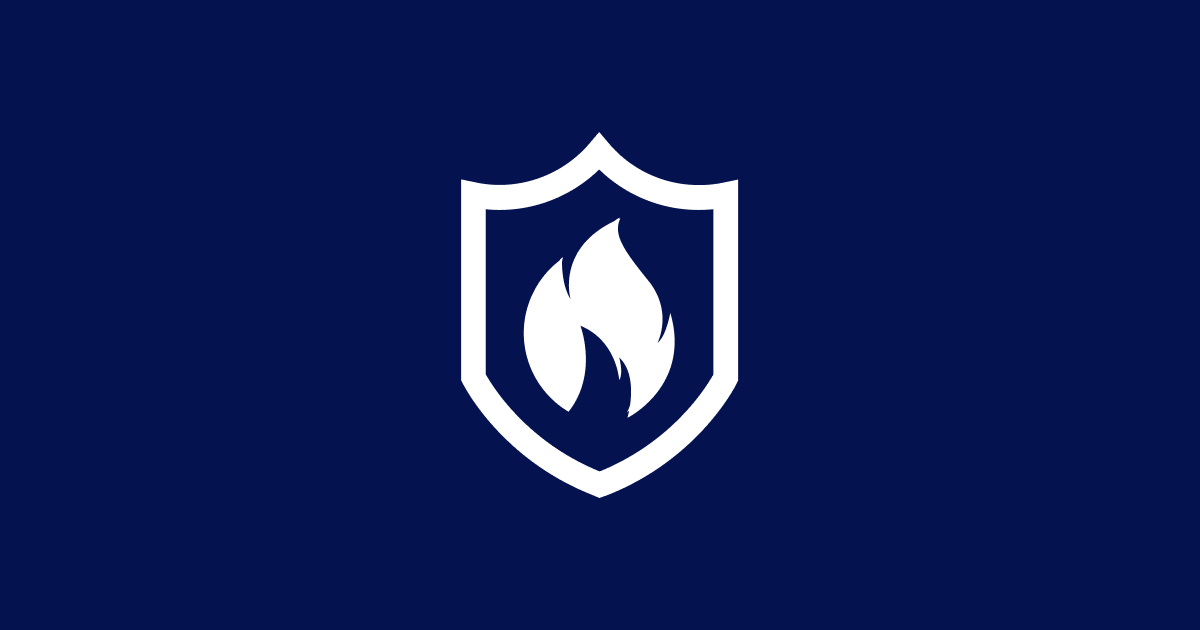
Implementing Mozilla's recommended cipher suites and TLS/SSL versions on node & npm
Improve your Node security with this configuration.
July 12, 2021
In our previous article, we discussed how using the iojs ciphers improves vanilla node 0.12. However Mozilla's Server Side TLS project also provides well thought out lists of cipher suites and TLS/SSL versions for a wide variety of servers.
The Mozilla settings provide 3 different levels:
- A better set of ciphers emphasizing GCM and TLS 1.2 exclusively for those who can handle IE11 up using the
modern
config. - Some slight more secure ciphers for IE7+ support using the
intermediate
config. - An
old
config, supporting IE6 upwards, only implemented in compatibility with the Mozilla tool. You really shouldn't be supporting IE6 for new web apps.
We implemented Mozilla's settings for ciphers and TLS/SSL versions in a npm module called ssl-config.
Usage:
Eg, for the 'modern' config:
var sslConfig = require('ssl-config')('modern');
Then run https.createServer
per node.js TLS and io.js TLS docs.
https.createServer({
key: privateKey,
cert: certificate,
ca: certificateAuthority,
ciphers: sslConfig.ciphers,
honorCipherOrder: true,
secureOptions: sslConfig.minimumTLSVersion
});
Comparing io.js settings and Mozilla
Mozilla emphasises GCM support - here is the 'modern' setting from Mozilla:
"ECDHE-RSA-AES128-GCM-SHA256",
"ECDHE-ECDSA-AES128-GCM-SHA256",
"ECDHE-RSA-AES256-GCM-SHA384",
"ECDHE-ECDSA-AES256-GCM-SHA384",
"DHE-RSA-AES128-GCM-SHA256",
"DHE-DSS-AES128-GCM-SHA256",
"kEDH+AESGCM",
"ECDHE-RSA-AES128-SHA256",
"ECDHE-ECDSA-AES128-SHA256",
"ECDHE-RSA-AES128-SHA",
"ECDHE-ECDSA-AES128-SHA",
"ECDHE-RSA-AES256-SHA384",
"ECDHE-ECDSA-AES256-SHA384",
"ECDHE-RSA-AES256-SHA",
"ECDHE-ECDSA-AES256-SHA",
"DHE-RSA-AES128-SHA256",
"DHE-RSA-AES128-SHA",
"DHE-DSS-AES128-SHA256",
"DHE-RSA-AES256-SHA256",
"DHE-DSS-AES256-SHA",
"DHE-RSA-AES256-SHA",
"!aNULL",
"!eNULL",
"!EXPORT",
"!DES",
"!RC4",
"!3DES",
"!MD5",
"!PSK"
Current io.js defaults are below. As previously mentioned, these are still much better than the default node 0.12 defaults:
"ECDHE-RSA-AES256-SHA384",
"DHE-RSA-AES256-SHA384",
"ECDHE-RSA-AES256-SHA256",
"DHE-RSA-AES256-SHA256",
"ECDHE-RSA-AES128-SHA256",
"DHE-RSA-AES128-SHA256",
"HIGH",
"!aNULL",
"!eNULL",
"!EXPORT",
"!DES",
"!RC4",
"!MD5",
"!PSK",
"!SRP",
"!CAMELLIA"
As you can see, AESGCM is preferred in the Mozilla ciphers. That's because GCM doesn't, as of the time of writing, have any known attacks.
A simpler way of setting minimum TLS/SSL versions
node and iojs normally set TLS versions using values from the constants
module, binary OR-ed with each other. Eg:
var constants = require('constants');
https.createServer({
...
// Set tls1.2 required by binary OR-ing every previous version of TLS/SSL
secureOptions: constants.SSL_OP_NO_TLSv1_1|constants.SSL_OP_NO_TLSv1|constants.SSL_OP_NO_SSLv3|constants.SSL_OP_NO_SSLv2)
});
If you like setting your ciphers manually, but simply want a nicer way to specify the minimum TLS/SSL version, we've also released minimum-tls-version for node. ssl-config uses it, but you can also use it directly:
var minimumTLSVersion = require('minimum-tls-version');
https.createServer({
...
secureOptions: mimumumTLSVersion('tlsv12')
});
Differences between Mozilla and the SSL Labs test.
The suite list uses the cipher suite prioritization logic from Mozilla. Right now, Firefox and Chrome don't support AES-GCM with 256 bit keys. A 128 bit AES key with GCM is considered superior to a 256 bit AES key with CBC.
SSL Labs, on the other hand, always prefers 256 bit keys. It's possible to remove the 128 bit keys and get a 100 for 'Cipher Strength' on SSL Labs test, however that will weaken your security and is thus A Bad Idea.
Note:
- This package only sets cipher suites and TLS/SSL versions, other parts of the recommendations are implemented elsewhere, eg, for Express servers HSTS we recommend using Helmet.
- This is not an official Mozilla project.
More info
For more information, visit ssl-config on npm.